A technique called recursion, a fascinating idea in computer programming, permits a function to call itself. We travel into a galaxy of recursive functions in this section of our Python crash course. Let’s examine the foundations, consider the idea’s potential, and discover how to use it successfully in Python.
Principles of Recursion
Recursion is the technique of solving a problem by breaking it down into more manageable, more compact instances. The issue gets a little bit closer to the basic situation, where it is simple to fix, with each recursive call. Consider it as a journey into the uncharted, where each step brings us a little bit nearer to the goal.
The Base Case: The Stopping Point
In any recursive function, the base case acts as the stopping point. It defines when the recursion should halt. Without a proper base case, a recursive function can run infinitely, consuming excessive memory. A well-defined base case is essential for the success of any recursive algorithm.
The Recursive Step: The Journey Continues
Once the base case is established, the recursive step is where the magic happens. It’s the moment when the function calls itself with a slightly modified version of the problem. This step takes us closer to the base case and, ultimately, to the solution.
A Simple Example: Calculating Factorials
Consider recursion in the context of calculating factorials, which is a well-known example. A non-negative integer’s factorial, denoted by the symbol n! is the sum of all positive numbers from 1 to n. n! = n * (n-1) * (n-2) *… * 2 * 1 is how this is expressed mathematically.
Recursive Function for Factorials:
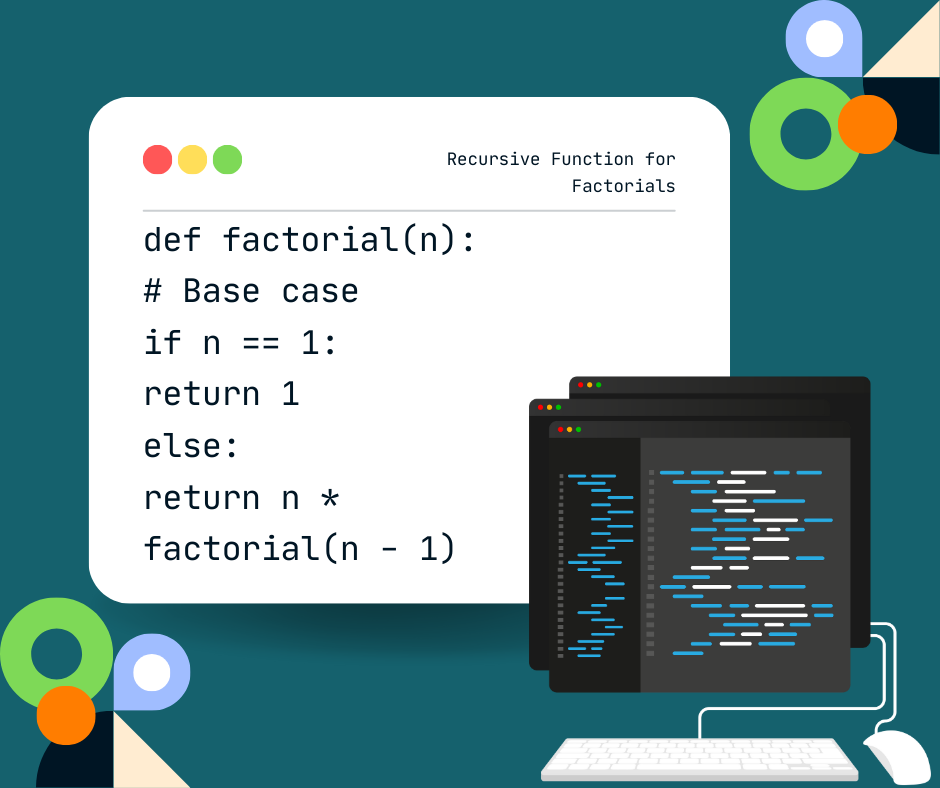
In Python, we may build a recursive function to compute factorials. Here is a simple example:
def factorial(n):
# Base case
if n == 1:
return 1
else:
return n * factorial(n – 1)
The Beauty of Recursion
Recursion is great because of how elegantly it handles challenging issues. We can approach a variety of issues elegantly and effectively by decomposing them into easier subproblems and making use of the power of recursive calls.
When to Use Recursion
Recursion is a powerful tool, but it isn’t always the best choice. It’s crucial to understand when to use recursion. Recursive solutions are often more concise and easier to understand for problems that exhibit a natural recursive structure, such as tree traversal or mathematical series. However, for problems with straightforward iterative solutions, recursion may introduce unnecessary complexity.